Building a Tic Tac Toe Game in Python: A Step-by-Step Guide
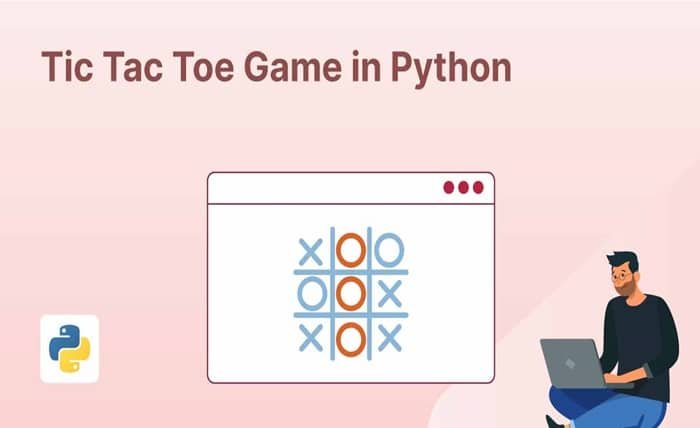
Tic Tac Toe is a classic game that many of us played as children. Building a Tic Tac Toe game in Python can be an excellent project for beginners looking to practice their coding skills. The simplicity of the game’s rules combined with the logical thinking required makes it an ideal project for learning Python. In this guide, we’ll walk through the process of creating a fully functioning Tic Tac Toe game in Python.
The Basics of Tic Tac Toe
Before we dive into coding the Tic Tac Toe game in Python, it’s important to understand the rules. Tic Tac Toe is played on a 3×3 grid, where two players take turns marking a space with either “X” or “O.” The objective of the game is to get three marks in a row, either horizontally, vertically, or diagonally. Our Python implementation will reflect these rules in a simple, logical manner.
Setting Up the Python Environment
To build the Tic Tac Toe game in Python, you’ll first need to set up your coding environment. If you haven’t already, install Python on your machine. You can use an IDE (Integrated Development Environment) like PyCharm, VSCode, or even a simple text editor like Sublime Text. Once you have Python set up, you’re ready to start writing the code for your Tic Tac Toe game in Python.
Creating the Tic Tac Toe Game Board in Python
The first step in building the Tic Tac Toe game in Python is creating the game board. In Python, we can represent the 3×3 grid as a list of lists. Here’s a simple way to create the board:
python
def create_board():
return [[' ' for _ in range(3)] for _ in range(3)]
This function will generate an empty Tic Tac Toe game board in Python, which we can later fill with “X” or “O” as the game progresses.
Displaying the Tic Tac Toe Game Board
Once we have our game board created, the next step is to display it so that players can see the current state of the Tic Tac Toe game in Python. We’ll create a function that prints the game board:
python
def display_board(board):
for row in board:
print('|'.join(row))
print('-' * 5)
This function will show the Tic Tac Toe game board in Python in a format that’s easy for players to understand.
Taking User Input in the Tic Tac Toe Game in Python
For the Tic Tac Toe game in Python to be interactive, we need to allow players to input their moves. We can use the input()
function in Python to capture user input. Here’s how we can implement it:
python
def player_move(board, symbol):
row = int(input("Enter row (0-2): "))
col = int(input("Enter column (0-2): "))
if board[row][col] == ' ':
board[row][col] = symbol
else:
print("This position is already taken.")
This function allows a player to input their move, and the Tic Tac Toe game in Python updates the board accordingly.
Checking for a Win Condition in the Tic Tac Toe Game in Python
A crucial part of any Tic Tac Toe game is determining whether a player has won. To check if there’s a winner in our Tic Tac Toe game in Python, we’ll need a function that evaluates the board after each move. Here’s a simple way to implement it:
python
def check_win(board, symbol):
for row in board:
if all([cell == symbol for cell in row]):
return True
for col in range(3):
if all([board[row][col] == symbol for row in range(3)]):
return True
if all([board[i][i] == symbol for i in range(3)]) or all([board[i][2-i] == symbol for i in range(3)]):
return True
return False
This function checks all possible winning conditions in the Tic Tac Toe game in Python: rows, columns, and diagonals.
Checking for a Draw in the Tic Tac Toe Game
In addition to checking for a win, we need to check if the Tic Tac Toe game in Python ends in a draw. A draw occurs when all spaces on the board are filled, and there is no winner. Here’s how we can implement this:
python
def check_draw(board):
for row in board:
if ' ' in row:
return False
return True
This function evaluates whether the Tic Tac Toe game in Python ends in a draw by checking if there are any empty spaces left.
Switching Between Players
In our Tic Tac Toe game in Python, two players take turns marking the board with either “X” or “O.” We’ll create a function to switch between players after each turn:
python
def switch_player(symbol):
return 'O' if symbol == 'X' else 'X'
This function will alternate between “X” and “O” to ensure that both players have a chance to play in the Tic Tac Toe game in Python.
The Main Game Loop
Now that we have the essential functions in place, we can create the main game loop for the Tic Tac Toe game in Python. This loop will continue running until there’s a win or a draw. Here’s the complete game loop:
python
def play_game():
board = create_board()
current_symbol = 'X'
while True:
display_board(board)
player_move(board, current_symbol)
if check_win(board, current_symbol):
display_board(board)
print(f"Player {current_symbol} wins!")
break
elif check_draw(board):
display_board(board)
print("It's a draw!")
break
current_symbol = switch_player(current_symbol)
This function combines everything we’ve built so far to create a fully functional Tic Tac Toe game in Python.
Enhancing the Tic Tac Toe Game in Python with AI
For those looking to take their Tic Tac Toe game in Python to the next level, implementing a simple AI is a great option. The AI can take the role of a player and make strategic moves. One basic approach is to randomly select an available spot:
python
import random
def ai_move(board, symbol):
available_moves = [(i, j) for i in range(3) for j in range(3) if board[i][j] == ‘ ‘]
move = random.choice(available_moves)
board[move[0]][move[1]] = symbol
This basic AI selects random empty spaces in the Tic Tac Toe game in Python, making the game more challenging for human players.
Optimizing the Tic Tac Toe Game with a Smarter AI
While the random AI works, we can create a smarter AI for our Tic Tac Toe game in Python by implementing the Minimax algorithm. The Minimax algorithm is designed to minimize the potential loss for a player, ensuring that the AI plays the best possible move.
python
def minimax(board, depth, is_maximizing):
if check_win(board, 'O'):
return 1
if check_win(board, 'X'):
return -1
if check_draw(board):
return 0
if is_maximizing:best_score = –float(‘inf’)
for i in range(3):
for j in range(3):
if board[i][j] == ‘ ‘:
board[i][j] = ‘O’
score = minimax(board, depth + 1, False)
board[i][j] = ‘ ‘
best_score = max(score, best_score)
return best_score
else:
best_score = float(‘inf’)
for i in range(3):
for j in range(3):
if board[i][j] == ‘ ‘:
board[i][j] = ‘X’
score = minimax(board, depth + 1, True)
board[i][j] = ‘ ‘
best_score = min(score, best_score)
return best_score
This implementation ensures that the AI in your Tic Tac Toe game in Python plays optimally, leading to a more competitive experience.
Conclusion
Creating a Tic Tac Toe game in Python is not only a fun project but also an excellent way to practice coding, problem-solving, and algorithm design. By breaking down the project into smaller tasks like creating the board, checking for win conditions, and implementing AI, you can build a fully functional Tic Tac Toe game in Python that both beginners and experienced programmers can enjoy. Whether you’re writing a simple two-player game or adding AI for a solo challenge, the Tic Tac Toe game in Python offers endless possibilities for learning and growth.
FAQs
1. How can I make the Tic Tac Toe game in Python more challenging?
You can add an AI opponent using algorithms like Minimax to make the Tic Tac Toe game in Python more challenging.
2. Is the Tic Tac Toe game in Python suitable for beginners?
Yes, building a Tic Tac Toe game in Python is a great project for beginners to practice basic programming concepts like loops, conditionals, and functions.
3. How do I implement a scoreboard in my Tic Tac Toe game in Python?
You can implement a scoreboard by adding variables to track the wins for each player and updating them after each game.
4. Can I add a graphical user interface (GUI) to my Tic Tac Toe game in Python?
Yes, you can use libraries like Tkinter or Pygame to add a GUI to your Tic Tac Toe game in Python.
5. How do I ensure the Tic Tac Toe game in Python doesn’t crash due to invalid input?
You can add error handling using try-except blocks and input validation to ensure the Tic Tac Toe game in Python handles invalid input gracefully.